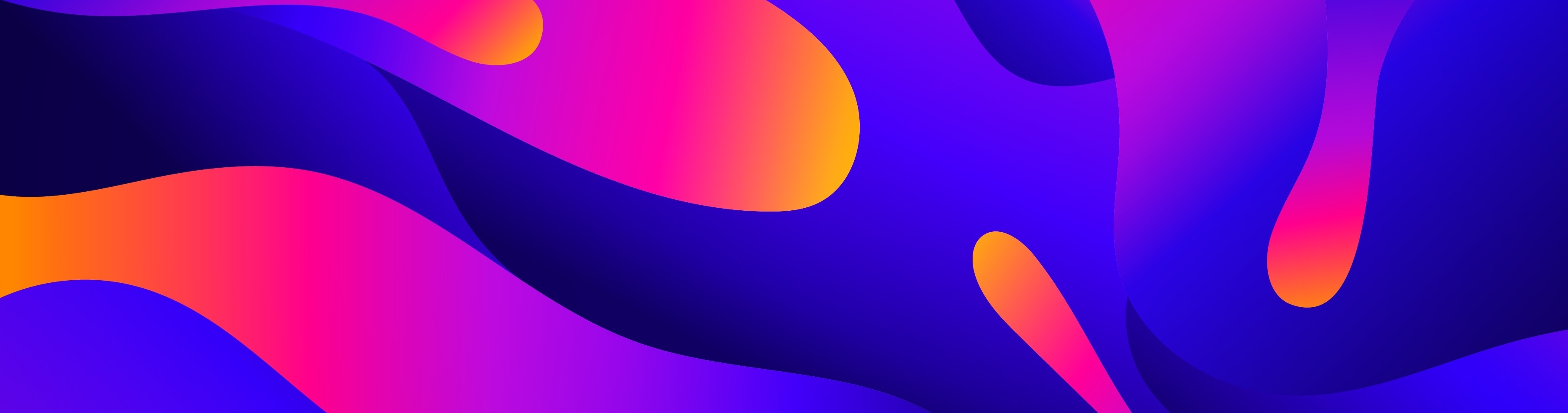
Boost your app’s usability with home screen widgets
In today's fast-paced digital world, mobile users demand quick, easy access to the information they care about most. Home screen widgets provide the perfect solution, offering bite-sized, interactive tools that provide instant access to important app features without opening the app itself. In our blog, we explore how home screen widgets boost user engagement, their various possibilities, and how to implement them across platforms like Flutter, iOS, and Android. Keep reading to discover how widgets can elevate your app’s visibility and user experience.
What is a home screen widget?
A homescreen widget is a small, interactive feature that sits on your phone's home screen, giving you quick access to useful information or app functions without having to open the app itself. Think of it as a mini version of an app that updates periodically and allows you to interact with it directly from the home screen—whether it’s checking the weather, viewing calendar events, or glancing at the latest news headlines.
These widgets are highly customizable, allowing users to arrange them as they like and interact with them effortlessly. By offering convenience at a glance, home screen widgets enhance the overall usability of a device without requiring users to dive into individual apps.
How home screen widgets increase user engagement
Widgets play a vital role in boosting user engagement by offering immediate access to an app’s core features. Here are a few ways they help:
- Instant access: Widgets provide quick, one-tap access to the most relevant features or information, saving users time and reducing the number of steps needed to complete an action.
- Visibility: Because they’re always visible on the home screen, widgets keep your app top of mind, leading to more frequent interactions and increased retention.
- Personalization: With increasing customizability options on both Android and iOS, integrating widgets allows users to personalize their phones, creating a more engaging experience.
- Increased user stickiness: By offering valuable content or functionality on the home screen, widgets make users more likely to stick with your app over time, as they become reliant on the convenience it offers.
- Real-time updates and notifications: Home screen widgets can provide real-time updates and notifications, keeping users engaged and returning to the app more frequently to check for the latest information.
By integrating widgets, you not only make your app more accessible but also increase the likelihood of users engaging with your app and content more frequently.
Different types of screen widgets
Home screen widgets vs lock screen widgets
While both home screen and lock screen widgets offer convenient access to information, they serve different purposes:
- Home screen widgets: These widgets on the home screen provide shortcuts to key app features without requiring users to open the app. Whether it’s weather updates, calendar events, or news headlines, home screen widgets are fully customizable and can be tailored to individual user preferences.
- Lock screen widgets: These widgets, available on iOS and Samsung devices, allow users to access essential notifications like calendar events or weather alerts without unlocking their phone. Although less customizable than home screen widgets, lock screen widgets still provide quick access to essential information or tools.
Informational widgets
Informational widgets provide users with at-a-glance information such as weather forecasts, calendar events, news headlines, or stock prices. They are designed to keep users informed without needing to open the corresponding app.
Interactive widgets
Interactive widgets go beyond just displaying information; they allow users to perform actions directly from the home screen. These can include features like buttons, sliders, or toggles. For example, an interactive widget for a smart home app could let users turn on the lights with a single tap—without opening the app.
Home screen widgets in Flutter
Flutter developers have a few options when it comes to implementing home screen widgets. You can opt for a complete native implementation, send data over a platform channel, or use a package to simplify the process.
Flutter implementation
When developing widgets for iOS, you must set up an app group to enable communication with the native iOS implementation. For a more streamlined Flutter integration, the home_widget package is a helpful tool. It allows developers to easily send and update data for native implementations. While creating the widget UI directly in Flutter isn’t possible, you can render Flutter widgets as images for display within the native framework. However, keep in mind that this method doesn’t support interactive elements like buttons.
Even with the home_widget package, there’s still a significant amount of native work required, as Flutter doesn’t natively support home screen widgets. To use the package, follow the setup instructions provided in its documentation. Specifically, for iOS widgets, you’ll need to set the AppGroupId to enable communication with the native iOS implementation.
HomeWidget.setAppGroupId(<YOUR APP GROUP ID>);
Next, you can follow the steps to display data on the widget. This includes using methods like saveWidgetData to send data or renderFlutterWidget to render Flutter widgets as images for display.
await HomeWidget.saveWidgetData<String>(<KEY FOR YOUR DATA>, <DATA YOU WANT TO DISPLAY>);
await HomeWidget.renderFlutterWidget(<FLUTTER WIDGET>, key: <KEY FOR YOUR DATA>, logicalSize: <SIZE OF YOUR IMAGE>, pixelRatio: <PIXEL RATIO>);
Remember that each time you make changes to your data, you must call the updateWidget method from the home_widget package to reflect those updates on the widget.
HomeWidget.updateWidget(
iOSName: <NAME OF YOUR IOS WIDGET>,
androidName: <NAME OF YOUR ANDROID WIDGET>,
qualifiedAndroidName: <QUALIFIED NAME OF YOUR ANDROID WIDGET>);
Home screen widgets in iOS
To implement home screen widgets in iOS, you'll need to create a widget extension and link both your app and the WidgetExtension to the same app group. This setup will automatically generate the new widget extension with all the required configurations.
To access the data sent via the home_widget package, retrieve it from UserDefaults. Create a UserDefaults object using the correct AppGroupId, then access the data using the same key you used in the saveWidgetData or renderFlutterWidget methods. For example, to retrieve a string titled "title," you would use the following:
let userDefaults = UserDefaults(suiteName: <YOUR APP GROUP>)
let title = userDefaults?.string(forKey: "title") ?? "No Title Set"
For more in-depth guidance on creating a home screen widget for iOS, consult the official documentation here.
Capabilities
Widgets in iOS come in multiple sizes, including small (one cell), medium (2x2 cells), and large (2 cells tall by 4 cells wide). Additionally, they can be customized, allowing users to adjust settings like a zip code for weather updates, select from predefined filters, or use a simple text field to modify the widget’s title.
Home screen widgets in Android
To create a home screen widget for an Android application, simply add an app widget to your Android project in Android Studio. This will automatically generate all the essential files needed for a basic home screen widget.
To access data sent via the home_widget package in Android, use the HomeWidgetPlugin. After importing the plugin, create a HomeWidgetPlugin object and use the getData method to retrieve the data:
import es.antonborri.home_widget.HomeWidgetPlugin;
// Retrieving data
Bundle widgetData = homeWidgetPlugin.getData(context);
// Extracting title
String title = widgetData.getString("title", null);
For more in-depth guidance on creating a home screen widget for Android, consult the official documentation here.
Capabilities
Android widgets offer a range of capabilities, including full resizability with customizable minimum and maximum width and height. Additionally, widgets can be made editable, allowing users to set various filter options, such as a zip code for a weather widget or a list of predefined filters. This flexibility also includes features like a simple text field that can dynamically update the widget's title.
Background tasks
To keep your widget updated, you can implement background tasks that periodically fetch data from the app, even when it’s not in use. In Flutter, this can be achieved using the workmanager package. However, if your widget’s UI is created in Flutter and rendered as images, it cannot be updated without reopening the app.
For iOS setup, start by enabling background fetch or background processing in your app's settings. Then, add the following code to the AppDelegate.swift file:
WorkmanagerPlugin.setPluginRegistrantCallback { registry in
GeneratedPluginRegistrant.register(with: registry)
}
UIApplication.shared.setMinimumBackgroundFetchInterval(TimeInterval(60*15))
This setup initiates a periodic data fetch that runs approximately every 15 minutes, though it may sometimes take longer.
In Flutter, begin by creating an entry point using the @pragma('vm:entry-point') annotation above your method in the main file. Use the executeTask method from the WorkManager class to filter specific tasks and run the necessary code to update your widget’s data. After defining the entry point, initialize it when the app starts. Then, register the periodic task, keeping in mind that the frequency defaults to 15 minutes and cannot be set to a shorter interval:
Workmanager().initialize(callbackDispatcher, isInDebugMode: true);
Workmanager().registerPeriodicTask(
"updateWidget",
"updateWidget",
frequency: const Duration(minutes: 15),
);
@pragma('vm:entry-point')
void callbackDispatcher() {
Workmanager().executeTask((task, inputData) async {
switch (task) {
case Workmanager.iOSBackgroundTask:
// Code to fetch data
break;
case "updateWidgetTask":
// Code to fetch data
break;
}
return Future.value(true);
});
}
This setup ensures that your widget stays current with the latest data, enhancing its functionality and user experience.
Conclusion
In summary, home screen widgets and lock screen widgets are vital components of mobile app development, providing users with easy access to information and features, which can significantly enhance engagement.
Home screen widgets enable quick access to specific functionalities and information directly from the device's home screen, improving usability and interaction. Meanwhile, lock screen widgets deliver important notifications without the need for users to unlock their devices, adding an extra layer of convenience.
Incorporating both types of widgets into your mobile app can greatly enhance user experience and accessibility. Utilizing tools like the home_widget package in Flutter allows for smooth communication between Flutter and native code, making widget integration seamless and effective.
📱 Interested in integrating screen widgets into your mobile app?